3D
How to access 3D information in a NUKE scene.
Getting basic selection information
Currently there are two nodes that provide the geo knob required to retrieve a vertex selection:
the Viewer node
the GeoSelect node
To access the selection info of those nodes at the most basic level, you would do this:
geoSelectKnob1 = nuke.activeViewer().node()['geo']
geoSelectKnob2 = nuke.toNode('GeoSelect1')['geo']
viewerSel = geoSelectKnob1.getSelection() #SELECTION IN THE CURRENTLY ACTIVE VIEWER
geoSel = geoSelectKnob2.getSelection() #SELECTION SAVED IN GEOSELECT NODE
The above will return a list of points per object. Each point in that list carries it’s selection value (‘1.0’ being selected and ‘0.0’ being unselected). So with the above code, this will return the selection state of point index 540 in the first object saved in the GeoSelects geo knob:
geoSel[0][540]
To get useful information from this:
def selectedVertexInfos(selectionThreshold=0.5):
'''
selectedPoints(selectionThreshold) -> iterator
Return an iterator which yields a tuple of the index and position of each point currently selected in the Viewer in turn.
The selectionThreshold parameter is used when working with a soft selection.
Only points with a selection level >= the selection threshold will be returned by this function.
'''
if not nuke.activeViewer():
return
geoSelectKnob = nuke.activeViewer().node()['geo']
sel = geoSelectKnob.getSelection()
objs = geoSelectKnob.getGeometry()
for o in xrange(len(sel)):
objSelection = sel[o]
objPoints = objs[o].points()
objTransform = objs[o].transform()
for p in xrange(len(objSelection)):
value = objSelection[p]
if value >= selectionThreshold:
pos = objPoints[p]
tPos = objTransform * _nukemath.Vector4(pos.x, pos.y, pos.z, 1.0)
yield VertexInfo(p, value, _nukemath.Vector3(tPos.x, tPos.y, tPos.z))
And with the above we can now get the vertex info of all selected points:
def getSelection(selectionThreshold=0.5):
# Build a VertexSelection from VertexInfos
vertexSelection = VertexSelection()
for info in selectedVertexInfos(selectionThreshold):
vertexSelection.add(info)
return vertexSelection
This may seem like an awful lot of hassle, which is why the nukescripts.snap3d module is provided to do all the above work for you for common tasks:
Working with the current selection
The easiest way to get information from the current vertex selection is using the nukescripts.snap3d module.:
for v in nukescripts.snap3d.getSelection():
print v
This will return the VertexInfo objects. To get each selected vertex’ position:
for v in nukescripts.snap3d.getSelection():
print v.position
There are many functions that will get the selection for you and do fancy stuff with it already. Here is a very simple example of how create a card at the selected vertices’ position and bind a hotkey to it:
nuke.menu( 'Viewer' ).addCommand( 'Quick Snap', lambda: nukescripts.snap3d.translateRotateToPoints( nuke.createNode( 'Card2' ) ), 'shift+q' )
All this is doing is calling the nukescripts.snap3d.translateRotateToPoints method on a newly created card and assigning this action to a menu and hotkey - done.
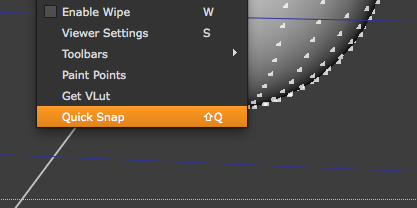
There are heaps of goodies in this module, so make sure to take a closer look:
dir( nukescripts.snap3d )
# Result:
['VertexInfo', 'VertexSelection', '__builtins__', '__doc__', '__file__', '__name__', '__package__', '_nukemath', 'addSnapFunc', 'allNodes', 'anySelectedPoint', 'averageNormal', 'calcAveragePosition', 'calcBounds', 'calcRotationVector', 'callSnapFunc', 'cameraProjectionMatrix', 'getSelection', 'math', 'nuke', 'planeRotation', 'projectPoint', 'projectPoints', 'projectSelectedPoints', 'rotateToPointsVerified', 'scaleToPointsVerified', 'selectedPoints', 'selectedVertexInfos', 'snapFuncs', 'translateRotateScaleSelectionToPoints', 'translateRotateScaleThisNodeToPoints', 'translateRotateScaleToPoints', 'translateRotateScaleToPointsVerified', 'translateRotateSelectionToPoints', 'translateRotateThisNodeToPoints', 'translateRotateToPoints', 'translateRotateToPointsVerified', 'translateSelectionToPoints', 'translateThisNodeToPoints', 'translateToPoints', 'translateToPointsVerified', 'transpose', 'verifyNodeOrder', 'verifyNodeToSnap', 'verifyVertexSelection']