Parameters and Knobs¶
A major feature of building compositing effects in Blink is providing parameters that allow artists to use and manipulate Blink effects without needing to understand code.
Kernel Parameters¶
The example kernel below defines parameters to generate all possible types of knobs.
The BlinkScript node will examine the kernel and generate UI knobs for all the parameters listed in the params
section (described here). These knobs will be listed to the Kernel Parameters tab and can then be controlled by the user, just like any other Nuke knobs.
Information such as a user-friendly label and default value are set in the define()
function (described here), through calls to defineParam()
.
// Copyright (c) 2024 The Foundry Visionmongers Ltd. All Rights Reserved.
/// Kernel demonstrating BlinkScript knob types
kernel Knobs : ImageComputationKernel<eComponentWise>
{
Image<eRead> src;
Image<eWrite> dst;
param:
float single_float;
float2 xy;
float3 xyz;
float4 acolor;
float multi_float[5];
float3x3 array3x3;
float4x4 array4x4;
int single_int;
int multi_int[4];
bool default_true_knob;
bool default_false_knob;
void define() {
// This knob will have a range of 0..8
defineParam(single_float, "SingleFloat", 4.0f);
defineParam(xy, "XY");
defineParam(xyz, "XYZ");
defineParam(acolor, "AColor");
defineParam(multi_float, "MultiFloat");
defineParam(array3x3, "Array3x3");
defineParam(array4x4, "Array4x4");
defineParam(single_int, "SingleInt", 4);
defineParam(multi_int, "MultiInt");
defineParam(default_true_knob, "DefaultTrue", true);
defineParam(default_false_knob, "DefaultFalse", false);
}
void process() {
// Just copy src to dst
dst() = src();
}
};
Knob Types¶
The following knob types can be generated by a Blink kernel.
Bool_knob

Parameters of type bool
will be represented by a Bool_knob, or single checkbox.
Int_knob

Parameters of type int
will generate an Int_knob with a single numeric input box. Right-clicking in the input box produces a menu which allows you to animate the value.
MultiInt_knob

Parameters of type int2
, int3
, int4
or int[]
will generate a MultiInt_knob, with multiple numeric input boxes. As with the Int_knob, right-clicking in the input boxes produces a menu which allows you to animate the values.
Float_knob

Parameters of type float
will generate a Float_knob with a numeric input box, linear slider and animation menu button. The slider will range from 0 to twice the default value of the parameter, or 0 to 1 if no default value has been set.
MultiFloat_knob

Parameters with numbers of floating-point values not already listed above, e.g. float[5]
, will generate a MultiFloat_knob, with a numerical entry box for each value, a single linear slider and an animation menu button. The slider will range from 0 to 1.
XY_knob

Parameters with two floating-point values, i.e. of type float2
or float[2]
, will be interpreted as positions in 2D space and will generate an XY_knob with a draggable handle in the Viewer.
XYZ_knob

Parameters with three floating-point values, i.e. of type float3
or float[3]
, will be interpreted as positions in 3D space and will generate an XYZ_knob with a draggable handle in the 3D Viewer.
AColor_knob
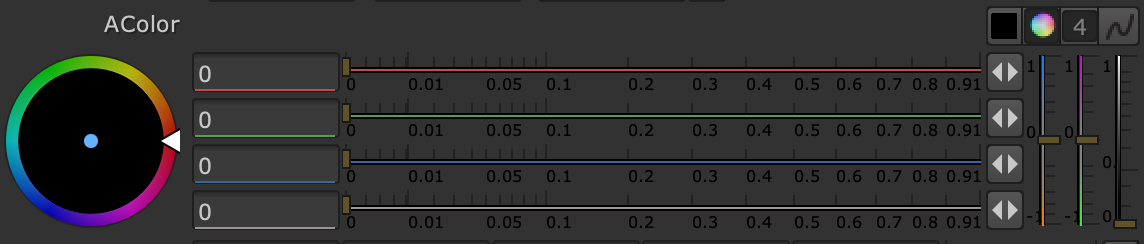
Parameters with four floating-point values, i.e. of type float4
or float[4]
, will be interpreted as colours with alpha, and will generate an AColor_knob. This initially shows a single entry box and slider, a button to split to four entry boxes, a button to pop up the Nuke color wheel, and a swatch showing the current color plus an eyedropper for sampling.
Array_knob
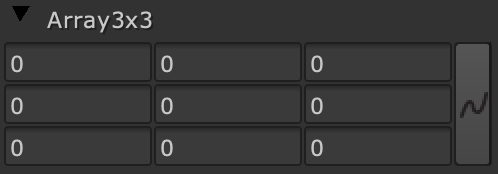
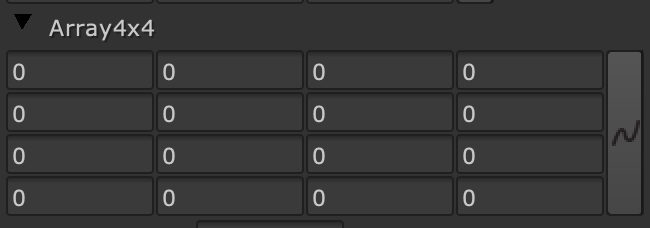
Parameters with nine floating-point values, including float3x3
, will be displayed as an Array_knob with a 3x3 grid. Parameters with sixteen floating-point values, including float4x4
, will be displayed as an Array_knob with a 4x4 grid.
Accessing Generated Knobs in Expressions¶
To create knob names for use in expressions, the BlinkScript node prepends the name of the kernel onto the display name for each of its generated knobs, after first removing unsupported characters such as spaces from the display name. For example, a parameter with the display name "Display Name"
, which is extracted from a kernel called MyKernel
, will be given the knob name MyKernel_DisplayName
.
To check the knob name for a knob you want to use in an expression, simply hover your mouse cursor over the knob’s input field; the first word that appears in bold will be the name of the knob.
Accessing Generated Knobs via Python Scripting¶
Once created, the knobs generated by the BlinkScript node are just the same as any other Nuke knobs and can be accessed via Python scripting. Python access requires the same knob names as are used in expressions (see Accessing Generated Knobs in Expressions), so to access the "Display Name"
parameter from kernel MyKernel
you would need to use MyKernel_DisplayName
.