Connecting Nodes
Adding and Removing Ports
Katana recipes are created by adding and connecting nodes in the Node Graph. Nodes are connected through their input and output ports. Some node types have a fixed number of ports, while others allow for an arbitrary number. The Merge nodes, for example, takes any number of inputs and combines them into a single output. To create a Merge node and add two input ports enter the following:
rootNode = NodegraphAPI.GetRootNode()
mergeNode = NodegraphAPI.CreateNode('Merge', rootNode)
firstPort = mergeNode.addInputPort("First")
secondPort = mergeNode.addInputPort("Second")
Ports can be added by index as well as by name, which allows direct control of their ordering. For example, to add an input port to the merge node created above, add the following:
newPort = mergeNode.addInputPortAtIndex("BetweenFirstAndSecond", 1)
- Node.addInputPort(name: str) Port
Creates and adds a new input port to this node with the given
name
, returning the port.Adds a
node_addInputPort
event to the event queue if the port was added.- Parameters:
name (
str
) – The name to attempt to use for the new input port.- Returns:
The created input port, whose name may differ from the given
name
.
- Node.addOutputPort(name: str) Port
Creates and adds a new output port to this node with the given
name
, returning the port.Adds a
node_addOutputPort
event to the event queue if the port was added.- Parameters:
name (
str
) – The name to attempt to use for the new output port.- Returns:
The created output port, whose name may differ from the given
name
.
- Node.addInputPortAtIndex(name: str, index: int) Port
Creates and adds a new input port to this node with the given
name
at the givenindex
, returning the port.Adds a
node_addInputPort
event to the event queue if the port was added.- Parameters:
name (
str
) – The name to attempt to use for the new input port.index (
int
) – The index at which to insert the new input port in the list of input ports.
- Returns:
The created input port, whose name may differ from the given
name
.
- Node.addOutputPortAtIndex(name: str, index: int) Port
Creates and adds a new output port to this node with the given
name
at the givenindex
, returning the port.Adds a
node_addOutputPort
event to the event queue if the port was added.- Parameters:
name (
str
) – The name to attempt to use for the new output port.index (
int
) – The index at which to insert the new output port in the list of output ports.
- Returns:
The created output port, whose name may differ from the given
name
.
- Node.removeInputPort(name: str) bool
Deletes an input port from this node by
name
, breaking any connections it had.Adds a
node_beginRemoveInputPort
event to the event quuee before disconnecting the port and attempting to remove it, and adds anode_removeInputPort
event to the event queue if the port was removed.- Parameters:
name (
str
) – The name of the input port to remove.- Returns:
True
if the input port was removed successfully, otherwiseFalse
.
- Node.removeOutputPort(name: str) bool
Deletes an output port from this node by
name
, breaking any connections it had.Adds a
node_removeOutputPort
event to the event queue if the port was removed.- Parameters:
name (
str
) – The name of the output port to remove.- Returns:
True
if the output port was removed successfully, otherwiseFalse
.
Retrieving Ports
- Node.getNumInputPorts() int
- Returns:
The number of input ports owned by this node.
- Node.getNumOutputPorts() int
- Returns:
The number of output ports owned by this node.
- Node.getInputPort(name: str) Port | None
- Parameters:
name (
str
) – The name of the input port to return.- Returns:
The input port from this node with the given
name
, orNone
if the port cannot be found.
- Node.getOutputPort(name: str) Port | None
- Parameters:
name (
str
) – The name of the output port to return.- Returns:
The output port from this node with the given
name
, orNone
if the port cannot be found.
- Node.getInputPortByIndex(index: int) Port
- Parameters:
index (
int
) – The index of the input port to return.- Returns:
The input port from this node with the given
index
, orNone
ifindex
is out of range.
- Node.getOutputPortByIndex(index: int) Port
- Parameters:
index (
int
) – The index of the output port to return.- Returns:
The output port from this node with the given
index
, orNone
ifindex
is out of range.
- Node.getInputPorts() list
- Returns:
A
list
of all input ports owned by this node.
- Node.getOutputPorts() list
- Returns:
A
list
of all output ports owned by this node.
- Node.getSourcePort(port: Port, graphState: GraphState | time: float) tuple
- Parameters:
port (
Port
) – The output port for which to return a source port.graphState (
GraphState
) – The Graph State context in which to obtain the source port.time (
float
) – The frame time at which to obtain the source port.
- Returns:
A 2-tuple of
(port, graphState)
or(port, time)
, withport
being the output port of this node that is used for producing the data of the givenport
in the context of the given Graph State or time. Here,port
will beNone
if not found.
- Node.getInputSource(name: str, graphState: GraphState | time: float) tuple
- Parameters:
name (
str
) – The name of the input port for which to return the input source.graphState (
GraphState
) – The Graph State context in which to evaluate the upstream nodes, determining the port routing e.g. of Switch nodes.time (
float
) – The frame time at which to evaluate the upstream nodes, determining the port routing e.g. of Switch nodes.
- Returns:
A 2-tuple of
(port, graphState}
or(port, time}
, whereport
is the output port of an upstream node that produces the scene for the input port with the givenname
of this node. Here,port
will beNone
if not found.
- Node.getInputPortAndGraphState(outputPort: Port, graphState: GraphState | time: float) tuple
Determines the input port (on the same node as the given
outputPort
) that is used when driving the output of that given output port. This is a similar function togetSourcePort()
, except that it doesn’t naturally recurse through the node graph - it returns the port on the same node as the given output port, if a logical input port is used. Similar togetSourcePort()
, returns the given output port in the case that no particular input port is in use as a source.For example, a VariableSwitch node returns the particular input port that is actively driving the given output port based on the Graph State provided.
getSourcePort()
with the same arguments recurses up through the node graph and return an output port.- Parameters:
outputPort (
Port
) – The output port for which to return a port.graphState (
GraphState
) – The Graph State context in which to obtain the port.time (
float
) – The frame time at which to obtain the port.
- Returns:
A 2-tuple of
(port, graphState)
or(port, time)
, withport
being the input port of this node that is used for producing the data of the givenoutputPort
in the context of the given Graph State or time. Here,port
will beNone
if not found.
Renaming Ports
The renameInputPort()
and renameOutputPort()
methods rename ports. For example, to rename the input port named “i0” and the
output port named “out” on the Merge node from earlier, enter the
following:
mergeNode.renameInputPort("i0", "input")
mergeNode.renameOutputPort("out", "output")
- Node.renameInputPort(oldName: str, newName: str) str | None
Renames the output port owned by this node from
oldName
tonewName
, returning the new unique name.Adds a
node_renameInputPort
event to the event queue if the port was renamed.- Parameters:
oldName (
str
) – The name of the input port to rename.newName (
str
) – The name to attempt to give to the input port.
- Returns:
The actual name that was set for the input port, which may differ from
newName
, as it needs to be unique, orNone
if no input port matches the givenoldName
.
- Node.renameOutputPort(oldName: str, newName: str) str | None
Renames the output port owned by this node from
oldName
tonewName
, returning the new unique name.Adds a
node_renameOutputPort
event to the event queue if the port was renamed.- Parameters:
oldName (
str
) – The name of the output port to rename.newName (
str
) – The name to attempt to give to the output port.
- Returns:
The actual name that was set for the output port, which may differ from
newName
, as it needs to be unique, orNone
if no output port matches the givenoldName
.
Connecting and Disconnecting Ports
The connect()
method links ports. For example, to take an output
port on a PrimitiveCreate node and connect it to an input port on a
Merge node enter the following:
primOutPort = primNode.getOutputPort("out")
mergeInPort = mergeNode.getInputPort("i0")
primOutPort.connect(mergeInPort)
# or...
mergeInPort.connect(primOutPort)
The disconnect()
method unlinks two ports. For example, to unlink
the two ports connected above enter the following:
mergeInPort.disconnect(primOutPort)
# or...
primOutPort.disconnect(mergeInPort)
- Port.isConnected(other: NodegraphAPI.Port) bool
Returns
True
if this port is connected to the given port.
- Port.getNumConnectedPorts() int
Returns the number of other ports connected to this port.
- Port.getConnectedPort(index: int) NodegraphAPI.Port
Returns the port connected to this port at the given
index
, orNone
ifindex
is out of range.
- Port.getConnectedPorts() list
Returns a
list
of all ports connected to this port.
- Port.connect(other: NodegraphAPI.Port, doCycleCheck: bool = True) bool
Connects
other
to this port. If the nodes to which the ports belong are not part of the same parent node, in-between connections along the way are created. ReturnsTrue
if the port connection was successfully established, orFalse
if recursion was detected, nodes were locked or if another error occurred.
- Port.disconnect(other: NodegraphAPI.Port) bool
Disconnects port
other
from this port. This does nothing if the ports are not directly connected. ReturnsTrue
if the ports were successfully disconnected.
Port Information
- Port.getIndex() int
Returns the index of this port inside its node.
- Port.getName() str
Returns the name that refers to this port.
- Port.getDisplayName(prefixWithPageNames: bool = True) str
Returns a UI-friendly name for this port that may consist of a label and the names of pages as defined in metadata on this port.
- Port.getType() int
Returns the type of this port, either
Port.Type_PRODUCER
orPort.TYPE_CONSUMER
.
Physical Source
A physical connection is determined by which output ports any given input port is directly connected. The physical connections are those you see represented by connection arrows in the Node Graph.
To find the physical source from a given port, use getConnectedPorts()
. For example, create a
PrimitiveCreate node and a Merge node, connect the output of the PrimitiveCreate node to an
input on the Merge node, then get the source of the connection into the Merge node:
# Get the root node
rootNode = NodegraphAPI.GetRootNode()
# Create a PrimitiveCreate node at root level
primNode = NodegraphAPI.CreateNode('PrimitiveCreate', rootNode)
# Create a Merge node at root level
mergeNode = NodegraphAPI.CreateNode('Merge', rootNode)
# Add an output port to the PrimitiveCreate node
primOutPort = primNode.addOutputPort("newOutputPort")
# Add an input to the Merge node
mergeInPort = mergeNode.addInputPort("fromPrim")
# Connect PrimitiveCreate to Merge
primOutPort.connect(mergeInPort)
# Use getConnectedPorts to find connections on mergeInPort
mergeInConnectedPorts = mergeInPort.getConnectedPorts()
# Print the connected port
print(mergeInConnectedPorts)
Logical Source
Logical connections are those used to traverse up the node graph at render time. Conditional logic, parameter values and time are used to determine the next relevant source of data, and this is represented by a logical connection between the current node and the next.
The diagram below shows an example of physical and logical connections in the Node Graph tab. Nodes A and B have physical connections to inputs on the Switch node, and node C has a physical connection to the output of the Switch.
The logical connection from node A or B to node C, depends on the setting of the switch. When the Switch node’s in parameter is set to 0, there is a logical connection from node A, to node C, passing through the Switch. When the Switch’s in parameter is set to 1, there is a logical connection from node B to node C, passing through the Switch.
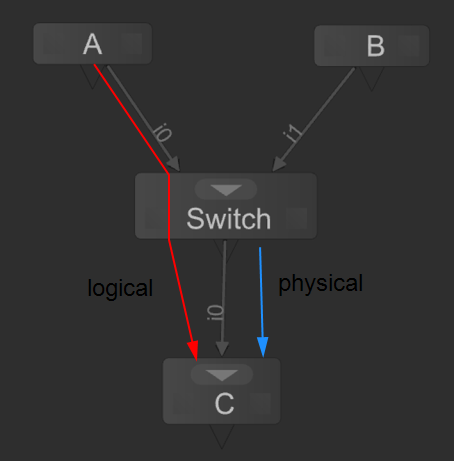
To find the logical source of an input node use getLogicalSource()
, which takes the name of the port and a
time as arguments, and returns a tuple containing the source port and the time. If no port is found, it returns None.
For example, recreate the scene shown above then find the input source at the output:
root = NodegraphAPI.GetRootNode()
# Create TheInputSourceNode at root level
primANode = NodegraphAPI.CreateNode('PrimitiveCreate', root)
primBNode = NodegraphAPI.CreateNode('PrimitiveCreate', root)
primANode.setName("A")
primBNode.setName("B")
primAOutPort = primANode.getOutputPort("out")
primBOutPort = primBNode.getOutputPort("out")
# Create the Switch node at root level
switchNode = NodegraphAPI.CreateNode('Switch', root)
switchInPort1 = switchNode.addInputPort("input1")
switchInPort2 = switchNode.addInputPort("input2")
switchOutPort = switchNode.getOutputPort("output")
# Create a Render node at root level
renderNode = NodegraphAPI.CreateNode('Render', root)
renderInPort = renderNode.getInputPort("input")
# Connect the primitive to the switch, and switch to render
primAOutPort.connect(switchInPort1)
primBOutPort.connect(switchInPort2)
switchOutPort.connect(renderInPort)
# Get the logical input of the render.input port
inputPort, inputTime = renderNode.getInputSource("input", TIME)
# Get hold of the source node so that we can print its name.
inputNode = inputPort.getNode()
inputNodeName = inputNode.getName()
# Print the name of the source node
print(inputNodeName)